Description
RESTinio is a header-only C++14 library that gives you an embedded HTTP/Websocket server. It is based on standalone version of ASIO and targeted primarily for asynchronous processing of HTTP-requests.
RESTinio alternatives and similar libraries
Based on the "Networking" category.
Alternatively, view RESTinio alternatives based on common mentions on social networks and blogs.
-
libcurl
A command line tool and library for transferring data with URL syntax, supporting DICT, FILE, FTP, FTPS, GOPHER, GOPHERS, HTTP, HTTPS, IMAP, IMAPS, LDAP, LDAPS, MQTT, POP3, POP3S, RTMP, RTMPS, RTSP, SCP, SFTP, SMB, SMBS, SMTP, SMTPS, TELNET, TFTP, WS and WSS. libcurl offers a myriad of powerful features -
POCO
The POCO C++ Libraries are powerful cross-platform C++ libraries for building network- and internet-based applications that run on desktop, server, mobile, IoT, and embedded systems. -
C++ REST SDK
The C++ REST SDK is a Microsoft project for cloud-based client-server communication in native code using a modern asynchronous C++ API design. This project aims to help C++ developers connect to and interact with services. -
RakNet
DISCONTINUED. RakNet is a cross platform, open source, C++ networking engine for game programmers. -
evpp
A modern C++ network library for developing high performance network services in TCP/UDP/HTTP protocols. -
Simple-Web-Server
DISCONTINUED. A very simple, fast, multithreaded, platform independent HTTP and HTTPS server and client library implemented using C++11 and Boost.Asio. Created to be an easy way to make REST resources available from C++ applications. -
wdt
DISCONTINUED. Warp speed Data Transfer (WDT) is an embeddedable library (and command line tool) aiming to transfer data between 2 systems as fast as possible over multiple TCP paths. -
PcapPlusPlus
PcapPlusPlus is a multiplatform C++ library for capturing, parsing and crafting of network packets. It is designed to be efficient, powerful and easy to use. It provides C++ wrappers for the most popular packet processing engines such as libpcap, Npcap, WinPcap, DPDK, AF_XDP and PF_RING. -
cpp-netlib
The C++ Network Library Project -- cross-platform, standards compliant networking library. -
Restbed
Corvusoft's Restbed framework brings asynchronous RESTful functionality to C++14 applications. -
Silicon
A high performance, middleware oriented C++14 http web framework please use matt-42/lithium instead -
Simple-WebSocket-Server
DISCONTINUED. A very simple, fast, multithreaded, platform independent WebSocket (WS) and WebSocket Secure (WSS) server and client library implemented using C++11, Boost.Asio and OpenSSL. Created to be an easy way to make WebSocket endpoints in C++. -
nope.c
WAFer is a C language-based software platform for scalable server-side and networking applications. Think node.js for C programmers. -
IXWebSocket
websocket and http client and server library, with TLS support and very few dependencies -
mailio
mailio is a cross platform C++ library for MIME format and SMTP, POP3 and IMAP protocols. It is based on standard C++ 17 and Boost library. -
QuantumGate
QuantumGate is a peer-to-peer (P2P) communications protocol, library and API written in C++. -
NetIF
Header-only C++14 library for getting addresses associated with network interfaces without name lookups on Windows, macOS, Linux, and FreeBSD
InfluxDB - Power Real-Time Data Analytics at Scale
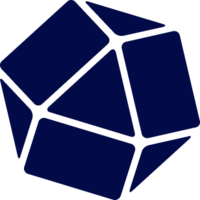
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of RESTinio or a related project?
README
What Is RESTinio?
RESTinio is a header-only C++14 library that gives you an embedded HTTP/Websocket server. It is based on standalone version of ASIO and targeted primarily for asynchronous processing of HTTP-requests. Since v.0.4.1 Boost::ASIO (1.66 or higher) is also supported (see notes on building with Boost::ASIO).
A Very Basic Example Of RESTinio
Consider the task of writing a C++ application that must support some REST API, RESTinio represents our solution for that task. Currently it is in stable beta state. Lets see how it feels like in the simplest case:
#include <restinio/all.hpp>
int main()
{
restinio::run(
restinio::on_this_thread()
.port(8080)
.address("localhost")
.request_handler([](auto req) {
return req->create_response().set_body("Hello, World!").done();
}));
return 0;
}
Server runs on the main thread, and respond to all requests with hello-world message. Of course you've got an access to the structure of a given HTTP request, so you can apply a complex logic for handling requests.
Features
- Async request handling. Cannot get the response data immediately? That's ok, store request handle somewhere and/or pass it to another execution context and get back to it when the data is ready.
- HTTP pipelining. Works well with async request handling. It might increase your server throughput dramatically.
- Timeout control. RESTinio can take care of bad connection that are like: send "GET /" and then just stuck.
- Response builders. Need chunked-encoded body - then RESTinio has a special response builder for you (obviously it is not the only builder).
- ExpressJS-like request routing (see an example below).
- An experimental typesafe request router that allows avoiding problems of ExpressJS-like router with help of static checks from C++ compiler.
- A possibility to chain several request-handlers (somewhat similar to ExpressJS's middleware).
- Working with query string parameters.
- Several ready-to-use helpers for working with HTTP headers (for example, the support for HTTP headers related to file uploading).
- Supports sending files and its parts (with sendfile on linux/unix and TransmitFile on windows).
- Supports compression (deflate, gzip).
- Supports TLS (HTTPS).
- Basic websocket support. Simply restinio::websocket::basic::upgrade() the request handle and start websocket session on a corresponding connection.
- Can run on external asio::io_context. RESTinio is separated from execution context.
- Some tune options. One can set acceptor and socket options. When running RESTinio on a pool of threads connections can be accepted in parallel.
Enhanced Example With Express Router
#include <restinio/all.hpp>
using namespace restinio;
template<typename T>
std::ostream & operator<<(std::ostream & to, const optional_t<T> & v) {
if(v) to << *v;
return to;
}
int main() {
// Create express router for our service.
auto router = std::make_unique<router::express_router_t<>>();
router->http_get(
R"(/data/meter/:meter_id(\d+))",
[](auto req, auto params) {
const auto qp = parse_query(req->header().query());
return req->create_response()
.set_body(
fmt::format("meter_id={} (year={}/mon={}/day={})",
cast_to<int>(params["meter_id"]),
opt_value<int>(qp, "year"),
opt_value<int>(qp, "mon"),
opt_value<int>(qp, "day")))
.done();
});
router->non_matched_request_handler(
[](auto req){
return req->create_response(restinio::status_not_found()).connection_close().done();
});
// Launching a server with custom traits.
struct my_server_traits : public default_single_thread_traits_t {
using request_handler_t = restinio::router::express_router_t<>;
};
restinio::run(
restinio::on_this_thread<my_server_traits>()
.address("localhost")
.request_handler(std::move(router)));
return 0;
}
License
RESTinio is distributed under BSD-3-CLAUSE license.
How To Use It?
The full documentation for RESTinio can be found here.
More
- Issues and bugs: Issue Tracker on GitHub.
- Discussion section on GitHub.
- Discussion group: restinio.
*Note that all licence references and agreements mentioned in the RESTinio README section above
are relevant to that project's source code only.