Simbody alternatives and similar libraries
Based on the "Physics" category.
Alternatively, view Simbody alternatives based on common mentions on social networks and blogs.
-
Bullet
Bullet Physics SDK: real-time collision detection and multi-physics simulation for VR, games, visual effects, robotics, machine learning etc. -
Jolt Physics
A multi core friendly rigid body physics and collision detection library, written in C++, suitable for games and VR applications. -
Newton Dynamics
Newton Dynamics is an integrated solution for real time simulation of physics environments.
InfluxDB - Power Real-Time Data Analytics at Scale
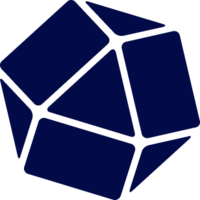
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of Simbody or a related project?
Popular Comparisons
README
Simbody

Simbody is a high-performance, open-source toolkit for science- and engineering-quality simulation of articulated mechanisms, including biomechanical structures such as human and animal skeletons, mechanical systems like robots, vehicles, and machines, and anything else that can be described as a set of rigid bodies interconnected by joints, influenced by forces and motions, and restricted by constraints. Simbody includes a multibody dynamics library for modeling motion in generalized/internal coordinates in O(n) time. This is sometimes called a Featherstone-style physics engine.
Simbody provides a C++ API that is used to build domain-specific applications; it is not a standalone application itself. For example, it is used by biomechanists in OpenSim, by roboticists in Gazebo, and for biomolecular research in MacroMoleculeBuilder (MMB). Here's an artful simulation of several RNA molecules containing thousands of bodies, performed with MMB by Samuel Flores:
[Sam Flores' Simbody RNA simulation][rna]
Read more about Simbody at the Simbody homepage.
Simple example: a double pendulum
Here's some code to simulate and visualize a 2-link chain:
#include "Simbody.h"
using namespace SimTK;
int main() {
// Define the system.
MultibodySystem system;
SimbodyMatterSubsystem matter(system);
GeneralForceSubsystem forces(system);
Force::Gravity gravity(forces, matter, -YAxis, 9.8);
// Describe mass and visualization properties for a generic body.
Body::Rigid bodyInfo(MassProperties(1.0, Vec3(0), UnitInertia(1)));
bodyInfo.addDecoration(Transform(), DecorativeSphere(0.1));
// Create the moving (mobilized) bodies of the pendulum.
MobilizedBody::Pin pendulum1(matter.Ground(), Transform(Vec3(0)),
bodyInfo, Transform(Vec3(0, 1, 0)));
MobilizedBody::Pin pendulum2(pendulum1, Transform(Vec3(0)),
bodyInfo, Transform(Vec3(0, 1, 0)));
// Set up visualization.
system.setUseUniformBackground(true);
Visualizer viz(system);
system.addEventReporter(new Visualizer::Reporter(viz, 0.01));
// Initialize the system and state.
State state = system.realizeTopology();
pendulum2.setRate(state, 5.0);
// Simulate for 20 seconds.
RungeKuttaMersonIntegrator integ(system);
TimeStepper ts(system, integ);
ts.initialize(state);
ts.stepTo(20.0);
}
[Double-pendulum simulation in Simbody][doublePendulum]
See Simbody's User Guide for a step-by-step explanation of this example.
Features
- Wide variety of joint, constraint, and force types; easily user-extended.
- Forward, inverse, and mixed dynamics. Motion driven by forces or prescribed motion.
- Contact (Hertz, Hunt and Crossley models).
- Gradient descent, interior point, and global (CMA) optimizers.
- A variety of numerical integrators with error control.
- Visualizer, using OpenGL
You want to...
- install Simbody.
- use Simbody in your own program.
- view API documentation.
- learn the theory behind Simbody.
- extend Simbody.
- get support at the Simbody Forum.
- report a bug or suggest a feature.
Dependencies
Simbody depends on the following:
- cross-platform building: CMake 2.8.10 or later (3.1.3 or later for Visual Studio).
- compiler: Visual Studio 2015, 2017, or 2019 (Windows only), gcc 4.9.0 or later (typically on Linux), Clang 3.4 or later, or Apple Clang (Xcode) 8 or later.
- linear algebra: LAPACK 3.6.0 or later and BLAS
- visualization (optional): FreeGLUT, Xi and Xmu
- API documentation (optional): Doxygen 1.8.6 or later; we recommend at least 1.8.8.
Using Simbody
- Creating your own Simbody-using project with CMake To get started with your own Simbody-using project, check out the [cmake/SampleCMakeLists.txt](cmake/SampleCMakeLists.txt) file.
Installing
Simbody works on Windows, Mac, and Linux. For each operating system, you can use a package manager or build from source. In this file, we provide instructions for 6 different ways of installing Simbody:
- Windows: build from source using Microsoft Visual Studio.
- Linux or Mac (make): build from source using gcc or Clang with make.
- Mac (Homebrew): automated build/install with Homebrew.
- Ubuntu/Debian: install pre-built binaries with apt-get.
- FreeBSD: install pre-built binaries with pkg.
- Windows using MinGW: build from source using MinGW.
- Windows/Mac/Linux: install pre-built binaries with the Conda package manager.
If you use Linux, check Repology to see if your distribution provides a package for Simbody.
These are not the only ways to install Simbody, however. For example, on a Mac, you could use CMake and Xcode.
C++11 and gcc/Clang
Simbody 3.6 and later uses C++11 features (the -std=c++11
flag). Simbody 3.3
and earlier use only C++03 features, and Simbody 3.4 and 3.5 can use either
C++03 or C++11; see the SIMBODY_STANDARD_11
CMake variable in these versions.
Note that if you want to use the C++11 flag in your own project, Simbody must
have been compiled with the C++11 flag as well.
Windows using Visual Studio
Get the dependencies
All needed library dependencies are provided with the Simbody installation on Windows, including linear algebra and visualization dependencies.
- Download and install Microsoft Visual Studio, version 2015, 2017, or 2019. The Community edition is free and sufficient.
- 2015: By default, Visual Studio 2015 does not provide C++ support; when installing, be sure to select Custom, and check Programming Languages > Visual C++ > Common Tools for Visual C++ 2015. If you have already installed Visual Studio without C++ support, simply re-run the installer and select Modify.
- 2017 and later: In the installer, select the Desktop development with C++ workload.
- Any other C++ code you plan to use with Simbody should be compiled with the same compiler as used for Simbody.
- Download and install CMake, version 3.1.3 or higher.
- (optional) If you want to build API documentation, download and install Doxygen, version 1.8.8 or higher.
Download the Simbody source code
- Method 1: Download the source code from https://github.com/simbody/simbody/releases. Look for the highest-numbered release, click on the .zip button, and unzip it on your computer. We'll assume you unzipped the source code into
C:/Simbody-source
. Method 2: Clone the git repository.
- Get git. There are many options:
- Git for Windows (most advanced),
- TortoiseGit (intermediate; good for TortoiseSVN users),
- GitHub Desktop (easiest).
- Get git. There are many options:
2. Clone the github repository into `C:/Simbody-source`. Run the following in a Git Bash / Git Shell, or find a way to run the equivalent commands in a GUI client:
$ git clone https://github.com/simbody/simbody.git C:/Simbody-source
$ git checkout Simbody-3.7
3. In the last line above, we assumed you want to build a released version.
Feel free to change the version you want to build.
If you want to build the latest development version ("bleeding edge") of
Simbody off the `master` branch, you can omit the `checkout` line.
To see the set of releases and checkout a specific version, you can use
the following commands:
$ git tag
$ git checkout Simbody-X.Y.Z
Configure and generate project files
- Open CMake.
- In the field Where is the source code, specify
C:/Simbody-source
. - In the field Where to build the binaries, specify something like
C:/Simbody-build
, just not inside your source directory. This is not where we will install Simbody; see below. - Click the Configure button.
- When prompted to select a generator, in the dropdown for Optional platform for generator, choose x64 to build 64-bit binaries or leave blank to build 32-bit binaries. In older versions of CMake, select a generator ending with Win64 to build 64-bit binaries (e.g., Visual Studio 14 2015 Win64 or Visual Studio 15 2017 Win64), or select one without Win64 to build 32-bit binaries (e.g., Visual Studio 14 2015 or Visual Studio 15 2017).
- Click Finish.
- Where do you want to install Simbody on your computer? Set this by changing the
CMAKE_INSTALL_PREFIX
variable. We'll assume you set it toC:/Simbody
. If you choose a different installation location, make sure to use yours where we useC:/Simbody
below. - Play around with the other build options:
BUILD_EXAMPLES
to see what Simbody can do. On by default.BUILD_TESTING
to ensure your Simbody works correctly. On by default.BUILD_VISUALIZER
to be able to watch your system move about! If building remotely, you could turn this off. On by default.BUILD_DYNAMIC_LIBRARIES
builds the three libraries as dynamic libraries. On by default. Unless you know what you're doing, leave this one on.BUILD_STATIC_LIBRARIES
builds the three libraries as static libraries, whose names will end with_static
. Off by default. You must activate eitherBUILD_DYNAMIC_LIBRARIES
,BUILD_STATIC_LIBRARIES
, or both.BUILD_TESTS_AND_EXAMPLES_STATIC
if static libraries, and tests or examples are being built, creates statically-linked tests/examples. Can take a while to build, and it is unlikely you'll use the statically-linked libraries.BUILD_TESTS_AND_EXAMPLES_SHARED
if tests or examples are being built, creates dynamically-linked tests/examples. Unless you know what you're doing, leave this one on.
- Click the Configure button again. Then, click Generate to make Visual Studio project files.
Build and install
- Open
C:/Simbody-build/Simbody.sln
in Visual Studio. Select your desired Solution configuration from the drop-down at the top.
- Debug: debugger symbols; no optimizations (more than 10x slower). Library and visualizer names end with
_d
. - RelWithDebInfo: debugger symbols; optimized. This is the configuration we recommend.
- Release: no debugger symbols; optimized. Generated libraries and executables are smaller but not faster than RelWithDebInfo.
- MinSizeRel: minimum size; optimized. May be slower than RelWithDebInfo or Release.
You at least want optimized libraries (all configurations but Debug are optimized), but you can have Debug libraries coexist with them. To do this, go through the full installation process twice, once for each configuration.
- Debug: debugger symbols; no optimizations (more than 10x slower). Library and visualizer names end with
Build the project ALL_BUILD by right-clicking it and selecting Build.
Run the tests by right-clicking RUN_TESTS and selecting Build. Make sure all tests pass. You can use RUN_TESTS_PARALLEL for a faster test run if you have multiple cores.
(Optional) Build the project doxygen to get API documentation generated from your Simbody source. You will get some warnings if your doxygen version is earlier than Doxygen 1.8.8; upgrade if you can.
Install Simbody by right-clicking INSTALL and selecting Build.
Play around with examples
Within your build in Visual Studio (not the installation):
- Make sure your configuration is set to a release configuration (e.g., RelWithDebInfo).
- Right click on one of the targets whose name begins with
Example -
and select Select as Startup Project. - Type Ctrl-F5 to start the program.
Set environment variables and test the installation
If you are only building Simbody to use it with OpenSim, you can skip this section.
- Allow executables to find Simbody libraries (.dll's) by adding the Simbody
bin/
directory to yourPATH
environment variable.- In the Start menu (Windows 7 or 10) or screen (Windows 8), search
environment
. - Select Edit the system environment variables.
- Click Environment Variables....
- Under System variables, click Path, then click Edit.
- Add
C:/Simbody/bin;
to the front of the text field. Don't forget the semicolon!
- In the Start menu (Windows 7 or 10) or screen (Windows 8), search
- Allow Simbody and other projects (e.g., OpenSim) to find Simbody. In the same Environment Variables window:
- Under User variables for..., click New....
- For Variable name, type
SIMBODY_HOME
. - For Variable value, type
C:/Simbody
.
- Changes only take effect in newly-opened windows. Close any Windows Explorer or Command Prompt windows.
- Test your installation by navigating to
C:/Simbody/examples/bin
and runningSimbodyInstallTest.exe
orSimbodyInstallTestNoViz.exe
.
Note: Example binaries are not installed for Debug configurations. They are present in the build environment, however, so you can run them from there. They will run very slowly!
Layout of installation
How is your Simbody installation organized?
bin/
the visualizer and shared libraries (.dll's, used at runtime).doc/
a few manuals, as well as API docs (SimbodyAPI.html
).examples/
src/
the source code for the examples.bin/
the examples, compiled into executables; run them! (Not installed for Debug builds.)
include/
the header (.h) files; necessary for projects that use Simbody.lib/
"import" libraries, used during linking.cmake/
CMake files that are useful for projects that use Simbody.
Linux or Mac using make
These instructions are for building Simbody from source on either a Mac or on Ubuntu.
Check the compiler version
Simbody uses recent C++ features, that require a modern compiler. Before installing Simbody, check your compiler version with commands like that:
g++ --version
clang++ --version
In case your compiler is not supported, you can upgrade your compiler.
Upgrading GCC to 4.9 on Ubuntu 14.04
Here are some instructions to upgrade GCC on a Ubuntu 14.04 distribution.
$ sudo add-apt-repository ppa:ubuntu-toolchain-r/test
$ sudo apt-get update
$ sudo apt-get install gcc-4.9 g++-4.9
If one wants to set gcc-4.9
and g++-4.9
as the default compilers, run the following command
$ sudo update-alternatives --install /usr/bin/gcc gcc /usr/bin/gcc-4.9 60 --slave /usr/bin/g++ g++ /usr/bin/g++-4.9
Remember that when having several compilers, CMake flags
CMAKE_C_COMPILER
and CMAKE_CXX_COMPILER
can be used
to select the ones desired. For example, Simbody can be
configured with the following flags:
$ cmake -DCMAKE_C_COMPILER=gcc-4.9 -DCMAKE_CXX_COMPILER=g++-4.9
Get dependencies
On a Mac, the Xcode developer package gives LAPACK and BLAS to you via the Accelerate framework. Mac's come with the visualization dependencies.
On Ubuntu, we need to get the dependencies ourselves. Open a terminal and run the following commands.
- Get the necessary dependencies:
$ sudo apt-get install cmake liblapack-dev
. - If you want to use the CMake GUI, install
cmake-qt-gui
. - For visualization (optional):
$ sudo apt-get install freeglut3-dev libxi-dev libxmu-dev
. - For API documentation (optional):
$ sudo apt-get install doxygen
.
LAPACK version 3.6.0 and higher may be required for some applications (OpenSim).
LAPACK can be downloaded from http://www.netlib.org/lapack/,
and compiled using the following method. It is sufficient to set LD_LIBRARY_PATH
to your LAPACK install prefix
and build Simbody using the -DBUILD_USING_OTHER_LAPACK:PATH=/path/to/liblapack.so
option in cmake.
cmake ../lapack-3.6.0 -DCMAKE_INSTALL_PREFIX=/path/to/new/lapack/ -DCMAKE_BUILD_TYPE=RELEASE -DBUILD_SHARED_LIBS=ON
make
make install
Get the Simbody source code
There are two ways to get the source code.
- Method 1: Download the source code from https://github.com/simbody/simbody/releases.
Look for the highest-numbered release, click on the .zip button, and unzip it on your computer.
We'll assume you unzipped the source code into
~/simbody-source
. Method 2: Clone the git repository.
- Get git.
- Mac: You might have it already, especially if you have Xcode, which
is free in the App Store. If not, one method is to install
Homebrew and run
brew install git
in a terminal. - Ubuntu: run
sudo apt-get install git
in a terminal.
- Mac: You might have it already, especially if you have Xcode, which
is free in the App Store. If not, one method is to install
Homebrew and run
Clone the github repository into
~/simbody-source
.$ git clone https://github.com/simbody/simbody.git ~/simbody-source $ git checkout Simbody-3.7
- Get git.
3. In the last line above, we assumed you want to build a released version.
Feel free to change the version you want to build.
If you want to build the latest development version ("bleeding edge") of
Simbody off the `master` branch, you can omit the `checkout` line.
To see the set of releases and checkout a specific version, you can use
the following commands:
$ git tag
$ git checkout Simbody-X.Y.Z
Configure and generate Makefiles
Create a directory in which we'll build Simbody. We'll assume you choose
~/simbody-build
. Don't choose a location inside~/simbody-source
.$ mkdir ~/simbody-build $ cd ~/simbody-build
Configure your Simbody build with CMake. We'll use the
cmake
command but you could also use the interactive toolsccmake
orcmake-gui
. You have a few configuration options to play with here.
* If you don't want to fuss with any options, run:
$ cmake ~/simbody-source
* Where do you want to install Simbody? By default, it is installed to `/usr/local/`. That's a great default option, especially if you think you'll only use one version of Simbody at a time. You can change this via the `CMAKE_INSTALL_PREFIX` variable. Let's choose `~/simbody`:
$ cmake ~/simbody-source -DCMAKE_INSTALL_PREFIX=~/simbody
* Do you want the libraries to be optimized for speed, or to contain debugger symbols? You can change this via the `CMAKE_BUILD_TYPE` variable. There are 4 options:
- **Debug**: debugger symbols; no optimizations (more than 10x slower). Library and visualizer names end with `_d`.
- **RelWithDebInfo**: debugger symbols; optimized. This is the configuration we recommend.
- **Release**: no debugger symbols; optimized. Generated libraries and executables are smaller but not faster than RelWithDebInfo.
- **MinSizeRel**: minimum size; optimized. May be slower than RelWithDebInfo or Release.
You at least want optimized libraries (all configurations but Debug are optimized),
but you can have Debug libraries coexist with them. To do this, go through
the full installation process twice, once for each configuration. It is
typical to use a different build directory for each build type (e.g.,
`~/simbody-build-debug` and `~/simbody-build-release`).
* There are a few other variables you might want to play with:
* `BUILD_EXAMPLES` to see what Simbody can do. On by default.
* `BUILD_TESTING` to ensure your Simbody works
correctly. On by default.
* `BUILD_VISUALIZER` to be able to watch your system
move about! If building on a cluster, you could turn this off. On by
default.
* `BUILD_DYNAMIC_LIBRARIES` builds the three libraries as dynamic libraries. On by default.
* `BUILD_STATIC_LIBRARIES` builds the three libraries as static libraries, whose names will end with `_static`.
* `BUILD_TESTS_AND_EXAMPLES_STATIC` if tests or examples are being built, creates statically-linked tests/examples. Can take a while to build, and it is unlikely you'll use the statically-linked libraries.
* `BUILD_TESTS_AND_EXAMPLES_SHARED` if tests or examples are being built, creates dynamically-linked tests/examples. Unless you know what you're doing, leave this one on.
You can combine all these options. Here's another example:
$ cmake ~/simbody-source -DCMAKE_INSTALL_PREFIX=~/simbody -DCMAKE_BUILD_TYPE=RelWithDebInfo -DBUILD_VISUALIZER=off
Build and install
Build the API documentation. This is optional, and you can only do this if you have Doxygen. You will get warnings if your doxygen installation is a version older than Doxygen 1.8.8.
$ make doxygen
Compile. Use the
-jn
flag to build usingn
processor cores. For example:$ make -j8
Run the tests.
$ ctest -j8
Install. If you chose
CMAKE_INSTALL_PREFIX
to be a location which requires sudo access to write to (like/usr/local/
, prepend this command with asudo
.$ make -j8 install
Just so you know, you can also uninstall (delete all files that CMake placed into CMAKE_INSTALL_PREFIX
) if you're in ~/simbody-build
.
$ make uninstall
Play around with examples
From your build directory, you can run Simbody's example programs. For instance, try:
$ ./ExamplePendulum
Set environment variables and test the installation
If you are only building Simbody to use it with OpenSim, you can skip this section.
Allow executables to find Simbody libraries (.dylib's or so's) by adding the Simbody lib directory to your linker path. On Mac, most users can skip this step.
If your
CMAKE_INSTALL_PREFIX
is/usr/local/
, run:$ sudo ldconfig
* If your `CMAKE_INSTALL_PREFIX` is neither `/usr/` nor `/usr/local/` (e.g., `~/simbody`'):
* Mac:
$ echo 'export DYLD_LIBRARY_PATH=$DYLD_LIBRARY_PATH:~/simbody/lib' >> ~/.bash_profile
* Ubuntu:
$ echo 'export LD_LIBRARY_PATH=$LD_LIBRARY_PATH:~/simbody/lib/x86_64-linux-gnu' >> ~/.bashrc
These commands add a line to a configuration file that is loaded every
time you open a new terminal. If using Ubuntu, you may need to replace
`x86_64-linux-gnu` with the appropriate directory on your computer.
Allow Simbody and other projects (e.g., OpenSim) to find Simbody. Make sure to replace
~/simbody
with yourCMAKE_INSTALL_PREFIX
.Mac:
$ echo 'export SIMBODY_HOME=~/simbody' >> ~/.bash_profile
Ubuntu:
$ echo 'export SIMBODY_HOME=~/simbody' >> ~/.bashrc
Open a new terminal.
Test your installation:
$ cd ~/simbody/share/doc/simbody/examples/bin $ ./SimbodyInstallTest # or ./SimbodyInstallTestNoViz
Layout of installation
The installation creates the following directories in CMAKE_INSTALL_PREFIX
. The directory [x86_64-linux-gnu]
only exists if you did NOT install to /usr/local/
and varies by platform. Even in that case, the name of your directory may be different.
include/simbody/
the header (.h) files; necessary for projects that use Simbody.lib/[x86_64-linux-gnu]/
shared libraries (.dylib's or .so's).cmake/simbody/
CMake files that are useful for projects that use Simbody.pkgconfig/
pkg-config files useful for projects that use Simbody.simbody/examples/
the examples, compiled into executables; run them! (Not installed for Debug builds.)
libexec/simbody/
thesimbody-visualizer
executable.share/doc/simbody/
a few manuals, as well as API docs (SimbodyAPI.html
).examples/src
source code for the examples.examples/bin
symbolic link to the runnable examples.
Mac and Homebrew
If using a Mac and Homebrew, the dependencies are taken care of for you.
Install
- Install Homebrew.
- Open a terminal.
Add the Open Source Robotics Foundation's list of repositories to Homebrew:
$ brew tap osrf/simulation
Install the latest release of Simbody.
$ brew install simbody
To install from the master branch instead, append
--HEAD
to the command above.
Where is Simbody installed?
Simbody is now installed to /usr/local/Cellar/simbody/<version>/
,
where <version>
is either the version number (e.g., 3.6.1
),
or HEAD
if you specified --HEAD
above.
Some directories are symlinked (symbolically linked) to /usr/local/
, which is where your system typically expects to find executables, shared libraries (.dylib's), headers (.h's), etc. The following directories from the Simbody installation are symlinked:
include/simbody -> /usr/local/include/simbody
lib -> /usr/local/lib
share/doc/simbody -> /usr/local/share/doc/simbody
Layout of installation
What's in the /usr/local/Cellar/simbody/<version>/
directory?
include/simbody/
the header (.h) files; necessary for projects that use Simbody.lib/
shared libraries (.dylib's), used at runtime.cmake/simbody/
CMake files that are useful for projects that use Simbody.pkgconfig/
pkg-config files useful for projects that use Simbody.simbody/examples/
the examples, compiled into executables; run them! (Not installed for Debug builds.)
libexec/simbody/
thesimbody-visualizer
executable.share/doc/simbody/
a few manuals, as well as API docs (SimbodyAPI.html
).examples/src
source code for the examples.examples/bin
symbolic link to executable examples.
Ubuntu and apt-get
Starting with Ubuntu 15.04, Simbody is available in the Ubuntu (and Debian) repositories. You can see a list of all simbody packages for all Ubuntu versions at the Ubuntu Packages website. The latest version of Simbody is usually not available in the Ubuntu repositories; the process for getting a new version of Simbody into the Ubuntu repositories could take up to a year.
Install
Open a terminal and run the following command:
$ sudo apt-get install libsimbody-dev simbody-doc
Layout of installation
Simbody is installed into the usr/
directory. The directory
[x86_64-linux-gnu]
varies by platform.
usr/include/simbody/
the header (.h) files; necessary for projects that use Simbody.usr/lib/[x86_64-linux-gnu]
shared libraries (.so's).cmake/simbody/
CMake files that are useful for projects that use Simbody.pkgconfig/
pkg-config files useful for projects that use Simbody.
usr/libexec/simbody/
thesimbody-visualizer
executable.usr/share/doc/simbody/
a few manuals, as well as API docs (SimbodyAPI.html
).examples/src
source code for the examples.examples/bin
symbolic link to executable examples.
FreeBSD and pkg
Simbody is available via the FreeBSD package repository.
Install
Open a terminal and run the following command:
$ sudo pkg install simbody
Windows using MinGW
Warning: The MinGW generation and build is experimental!
This build is still experimental, because of :
- the various MinGW versions available (Thread model, exception mechanism)
- the compiled libraries Simbody depends on (Blas, Lapack and optionnaly glut).
Below are three sections that gives a list of supported versions, command line instructions, and reasons why is it not so obvious to use MinGW.
Supported MinGW versions
If you do not want to go into details, you need a MinGW version with :
- a Posix thread model and Dwarf exception mechanism on a 32 bit computer
- a Posix thread model and SJLJ exception mechanism on a 64 bit computer
Other versions are supported with additional configurations.
The table below lists the various versions of MinGW versions tested:
OS | Thread | Exception | Comment | URL | |
---|---|---|---|---|---|
1 | 64 Bits | Posix | SJLJ | All features supported, all binary included (Recommended version) | MinGW64 GCC 5.2.0 |
2 | 64 Bits | Posix | SEH | Needs to be linked against user's Blas and Lapack | MinGW64 GCC 5.2.0 |
3 | 32 Bits | Posix | Dwarf | No visualization, all binary included | MinGW64 GCC 5.2.0 |
4 | 32 Bits | Posix | SJLJ | No visualization, needs to be linked against user's Blas and Lapack | MinGW64 GCC 5.2.0 |
We recommend to use the first configuration where all features are supported and
does not need additional libraries to compile and run.
The URL allows to download directly this version.
The second version needs to be linked against user's Blas and Lapack
(A CLI example is given below).
Blas and Lapack sources can be downloaded from
netlib.
For the 3rd and 4th versions that run that target a 32 bit behaviour,
visualization is not possible for the time being.
(It is due to a compile and link problem with glut
).
Moreover for the 4th one, one needs to provide Blas and Lapack libraries.
Please note that only Posix version of MinGW are supported.
If your version is not supported, CMake will detect it while configuring and stops.
Instructions
Below are some examples of command line instructions for various cases.
It is assumed you are running commands from a build directory, that can access Simbody source with a command cd ..\simbody
.
It is recommended to specify with the installation directory with flag CMAKE_INSTALL_PREFIX
(e.g. -DCMAKE_INSTALL_PREFIX="C:\Program Files\Simbody"
).
If not used, the installation directory will be C:\Program Files (x86)\Simbody
on a 64 bit computer. This might be confusing since it is the 32 bit installation location.
Example of instructions where one uses Blas and Lapack libraries provided (to be used in a Windows terminal, where MinGW is in the PATH):
rem CMake configuration
cmake ..\simbody -G "MinGW Makefiles" -DCMAKE_BUILD_TYPE=Release -DCMAKE_INSTALL_PREFIX="C:\Program Files\Simbody"
rem Compilation
mingw32-make
rem Test
mingw32-make test
rem Installation
mingw32-make install
Example of instructions where one uses Blas and Lapack libraries provided (to be used in a Windows terminal, where MinGW is NOT in the PATH):
rem Variable and path definition
set CMAKE="C:\Program Files\CMake\bin\cmake.exe"
set MinGWDir=C:\Program Files\mingw-w64\i686-5.2.0-posix-sjlj-rt_v4-rev0\mingw32
set PATH=%MinGWDir%\bin;%MinGWDir%\i686-w64-mingw32\lib
rem CMake configuration
%CMAKE% ..\simbody -G"MinGW Makefiles" -DCMAKE_BUILD_TYPE=Release ^
-DCMAKE_INSTALL_PREFIX="C:\Program Files\Simbody" ^
-DCMAKE_C_COMPILER:PATH="%MinGWDir%\bin\gcc.exe" ^
-DCMAKE_CXX_COMPILER:PATH="%MinGWDir%\bin\g++.exe" ^
-DCMAKE_MAKE_PROGRAM:PATH="%MinGWDir%\bin\mingw32-make.exe"
rem Compilation
mingw32-make
rem Test
mingw32-make test
rem Installation
mingw32-make install
Example of instructions where one uses Blas and Lapack libraries provided (to be used in a MSYS terminal with MinGW in the PATH):
# CMake configuration
cmake ../simbody -G "MSYS Makefiles" -DCMAKE_BUILD_TYPE=Release -DCMAKE_INSTALL_PREFIX="C:\Program Files\Simbody"
# Compilation
make
# Test
make test
# Installation
make install
Example of instructions where one provides our own Blas and Lapack libraries (to be used in a MSYS terminal with MinGW in the PATH):
# CMake configuration
cmake ../simbody -G"MSYS Makefiles" -DCMAKE_BUILD_TYPE=Release \
-DCMAKE_INSTALL_PREFIX="C:\Program Files\Simbody" \
-DCMAKE_C_COMPILER:PATH="C:\Program Files\mingw-w64\i686-5.2.0-posix-sjlj-rt_v4-rev0\mingw32\bin\gcc.exe" \
-DCMAKE_CXX_COMPILER:PATH="C:\Program Files\mingw-w64\i686-5.2.0-posix-sjlj-rt_v4-rev0\mingw32\bin\g++.exe" \
-DBUILD_USING_OTHER_LAPACK:PATH="C:\Program Files\lapack-3.5.0\bin\liblapack.dll;C:\Program Files\lapack-3.5.0\bin\libblas.dll"
make
# Test
make test
# Installation
make install
MinGW details
This paragraph explains the reason why one can not use any MinGW version.
MinGW is available with two thread models :
- Win32 thread model
- Posix thread model
One has to use the Posix thread model, since all thread functionalities (e.g. std:mutex
) are not implemented.
To ease building on Windows, Simbody provides compiled libraries for Blas and Lapack :
- On Windows 32 Bits, these were compiled with a Dwarf exception mechanism,
- On Windows 64 Bits, these were compiled with a SJLJ exception mechanism.
If one chooses a MinGW compilation, we need to respect this exception mechanism. A program can not rely on both mechanisms. This means that if we want to use the compiled libraries, our MinGW installation should have the same exception mechanism. Otherwise, we need to provide our own Blas and Lapack libraries.
To see which exception mechanism is used, user can look at dlls located in the bin
directory of MinGW.
The name of mechanism is present in the file libgcc_XXXX.dll
, where XXXX
can be dw
, seh
or sjlj
.
For some MinGW versions, this information is also available by looking at the result of gcc --version
.
CMake will check the version of your MinGW, and if the exception mechanism is different,
then the configuration stops because of this difference.
If one provides Blas and Lapack libraries with the CMake variable BUILD_USING_OTHER_LAPACK
,
compilation with MinGW is always possible.
Windows, Mac, and Linux Using Conda
Conda is a cross platform package manager that can
be used to install Simbody on Windows, Mac, or Linux. To install Simbody using
Conda you must first install
Miniconda or
Anaconda. Either of these will provide
the conda
command which can be invoked at the command line to install Simbody
from the Conda Forge channel as follows:
$ conda install -c conda-forge simbody
This command will install Simbody (both the libraries and headers) into the Miniconda or Anaconda installation directory as per the standard layout for each of the operating systems described above. The Conda Forge Simbody recipe can be found in Conda Forge's feedstock repository.
Acknowledgments
We are grateful for past and continuing support for Simbody's development in Stanford's Bioengineering department through the following grants:
- NIH U54 GM072970 (Simulation of Biological Structures)
- NIH U54 EB020405 (Mobilize Center)
- NIH R24 HD065690 (Simulation in Rehabilitation Research)
- OSRF subcontract 12-006 to DARPA HR0011-12-C-0111 (Robotics Challenge)
Prof. Scott Delp is the Principal Investigator on these grants and Simbody is used extensively in Scott's Neuromuscular Biomechanics Lab as the basis for the OpenSim biomechanical simulation software application for medical research.